Articles about: dotnet
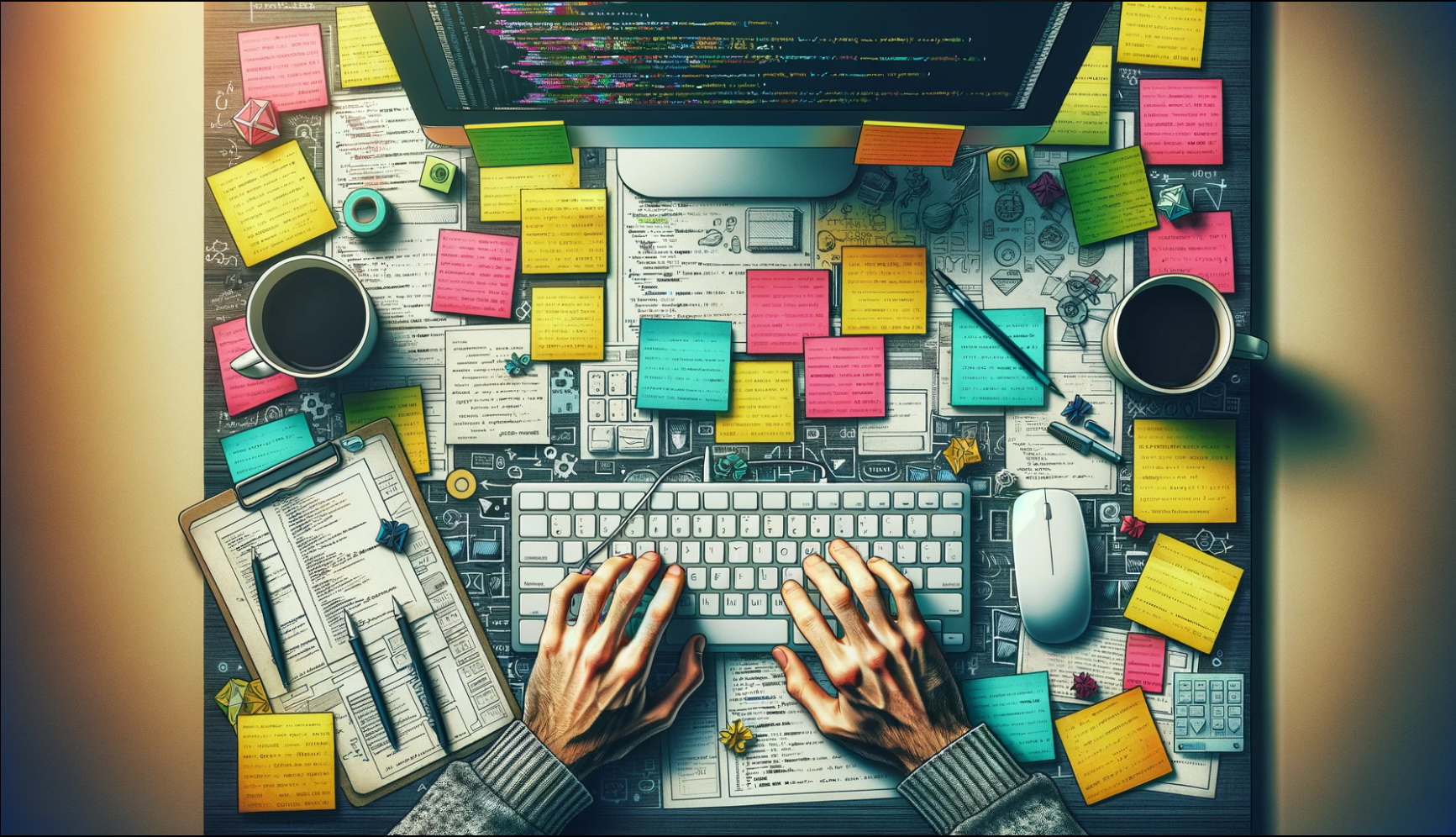
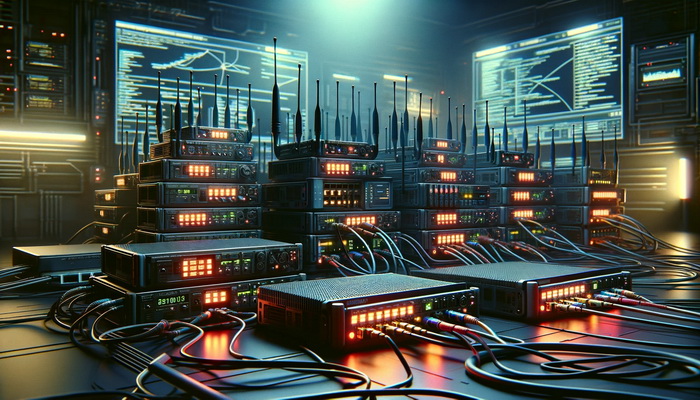
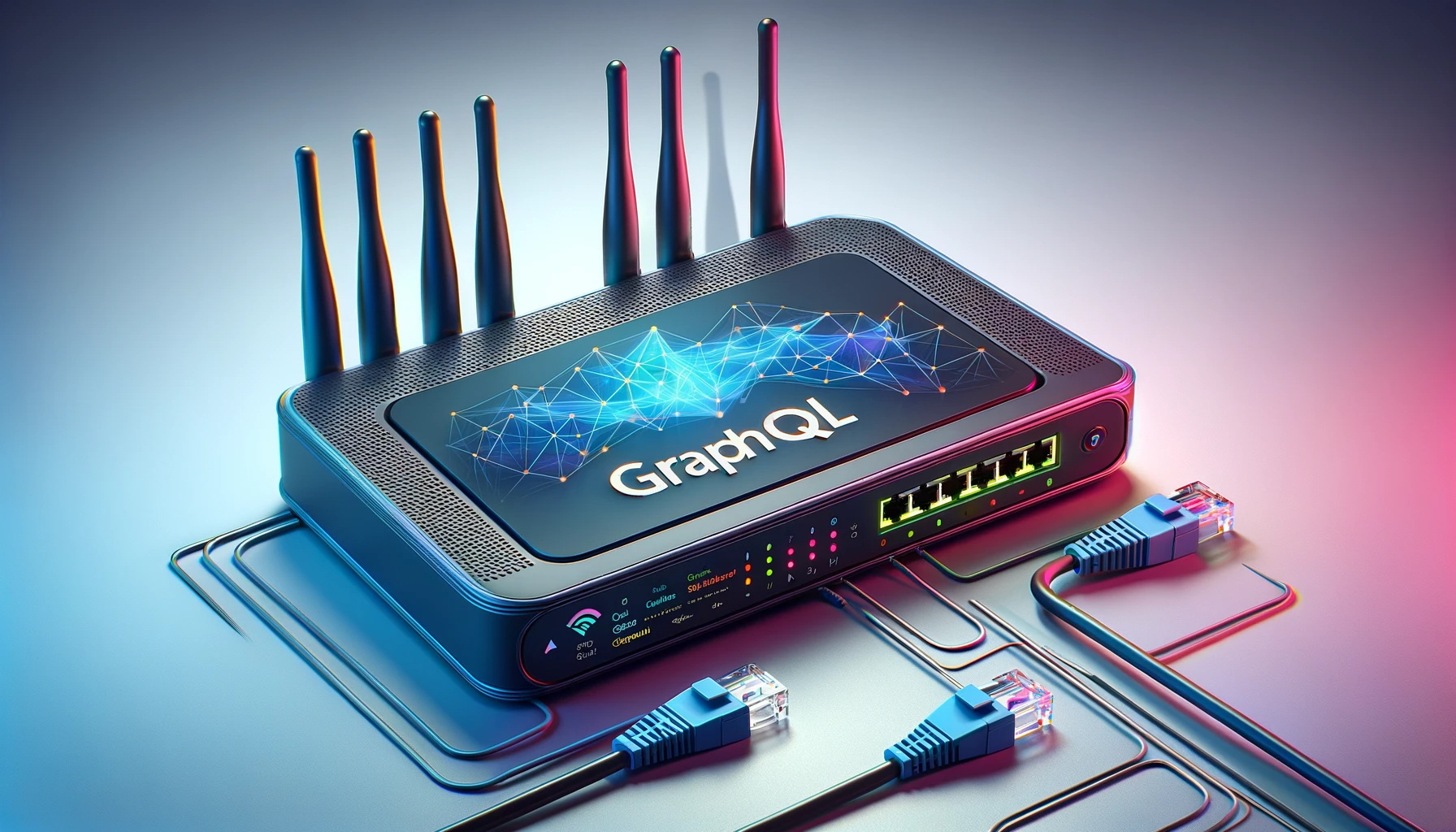
GraphQL is a query language for APIs that allows clients to request exactly what they need, making data retrieval more efficient than traditional REST APIs. It supports three different types of client-server interaction: queries, mutations and subscriptions. When you start integrating a GraphQL API as a consumer in your application, it’s likely that you’ll need to write automated tests to ensure that the integration works correctly. In this blog post, I will show you how to mock GraphQL queries using WireMock.NET.
... Read More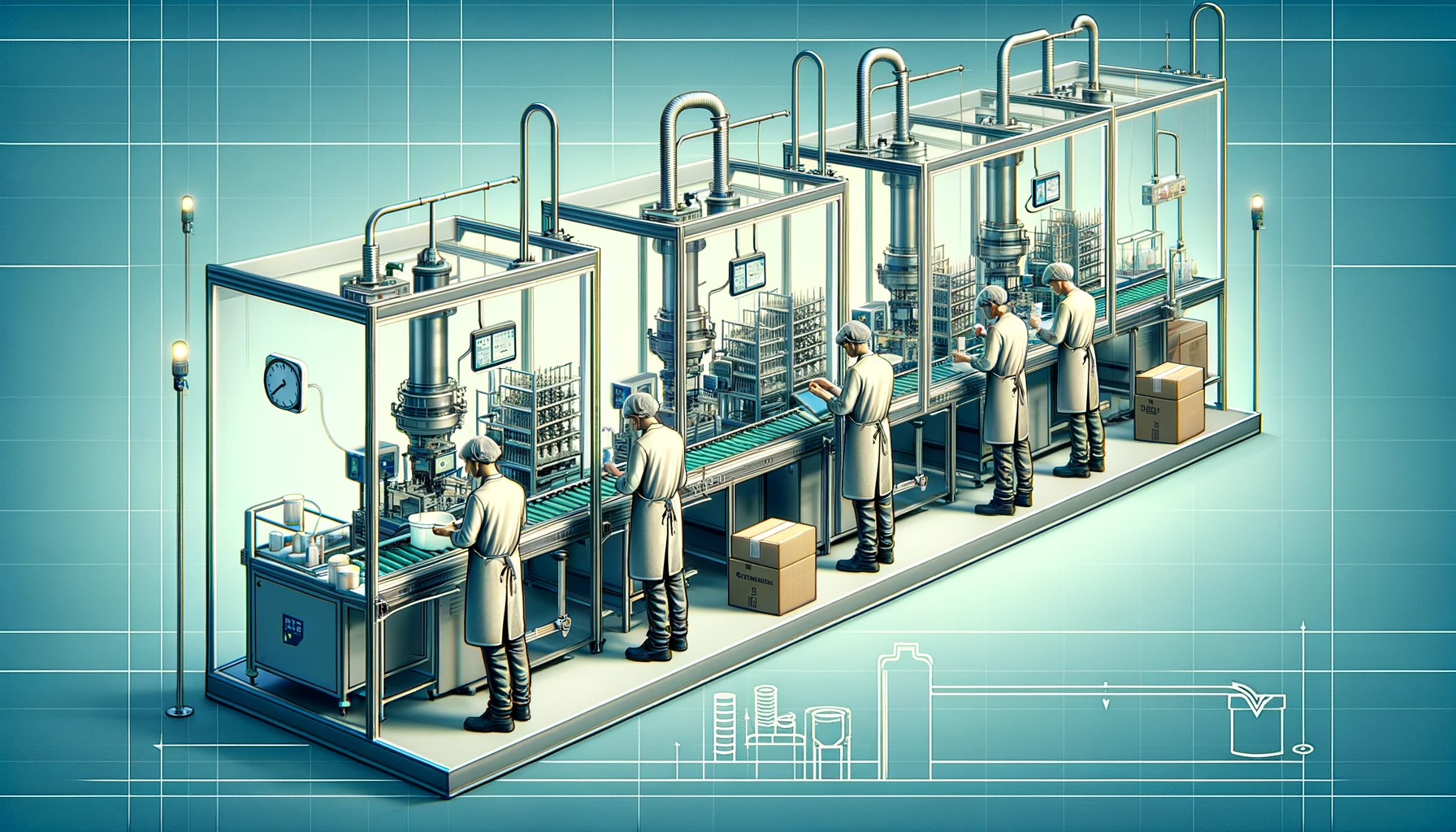
In this blog post I describe the typical problems caused by the usage of Setup
and Teardown
method in dotnet tests and how those problems can be solved by using only C# language features.
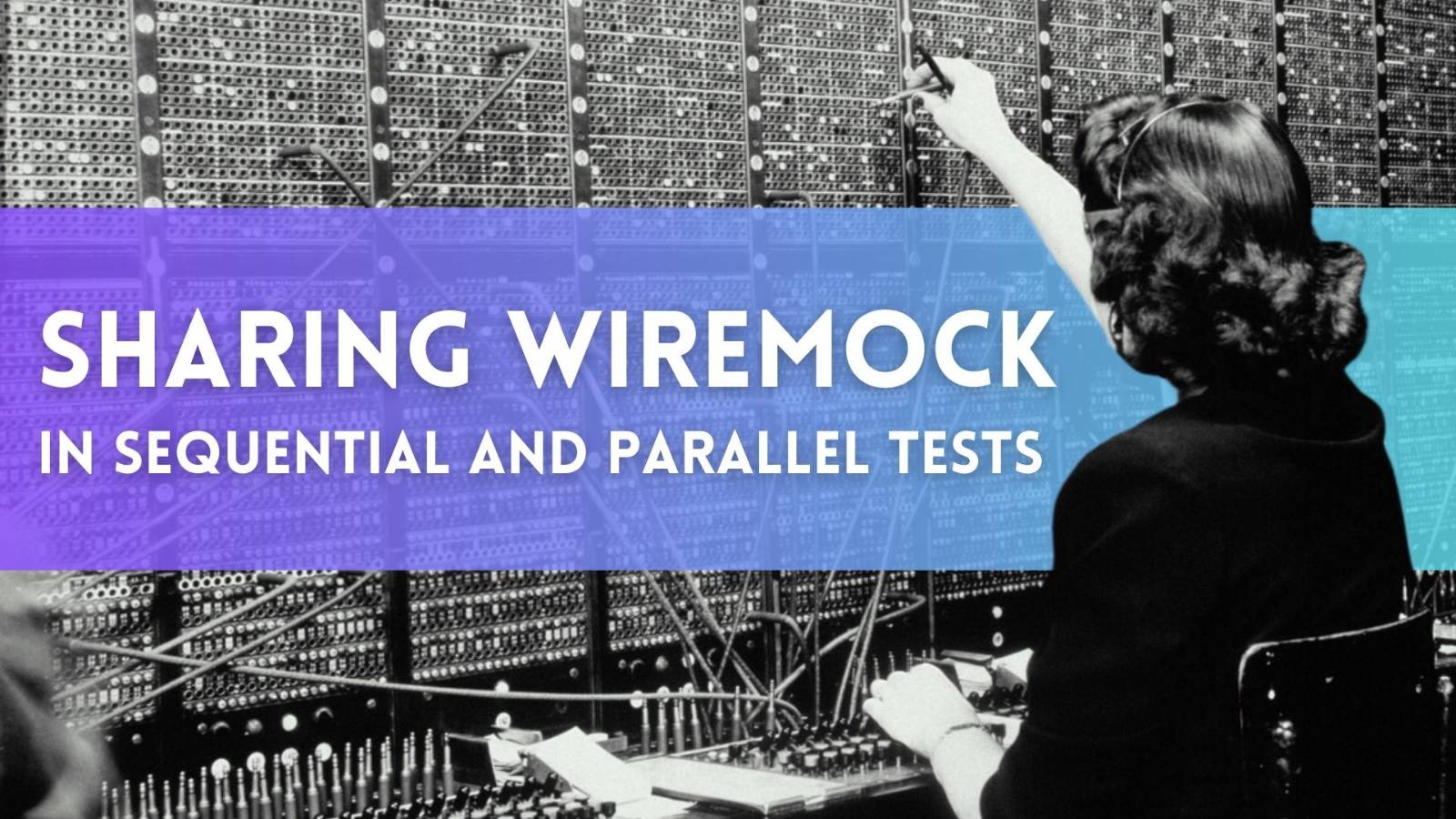
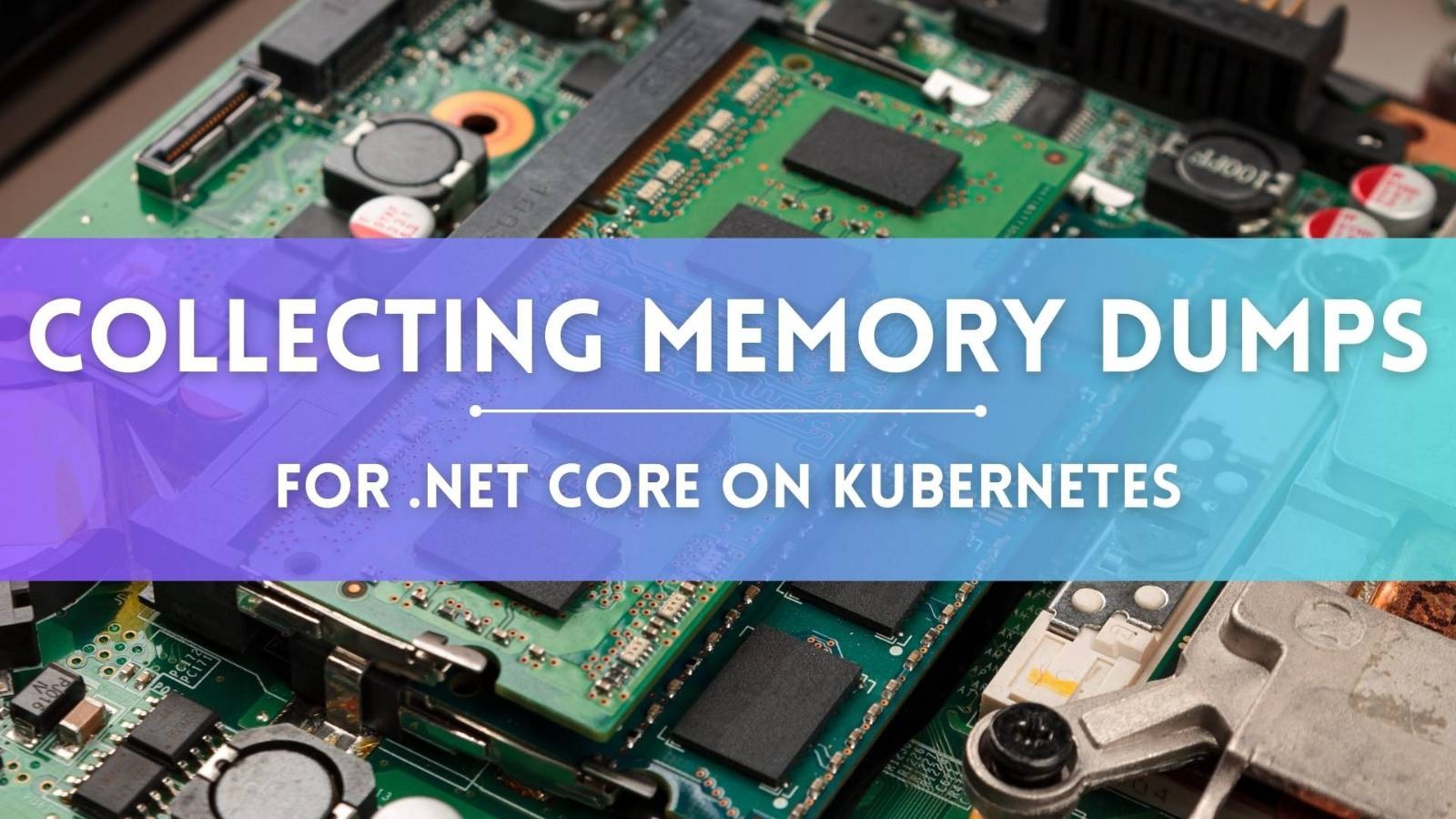
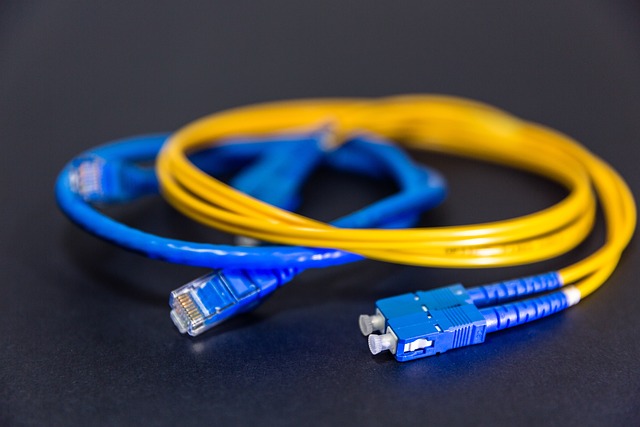
In the second installment of my blog post series on WireMock.NET, I will be discussing some of the most common problems that developers encounter while using the library. WireMock troubleshooting can be quite time consuming, especially when you don’t know the drill. I hope that my guideline will save you a lot of time.
... Read More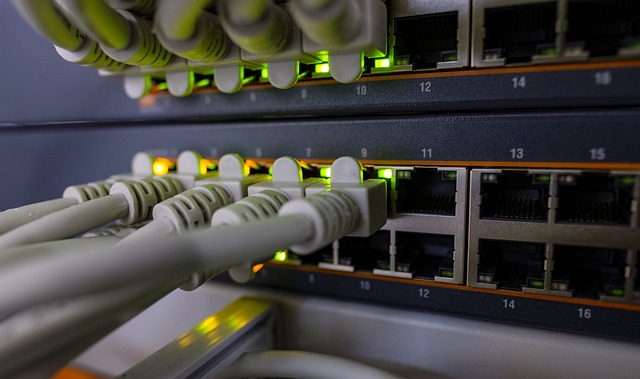
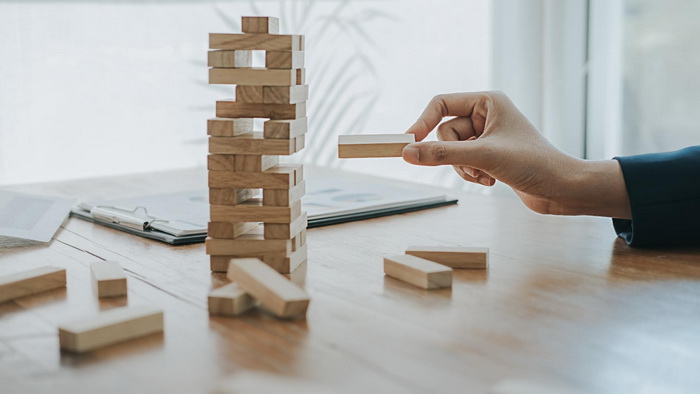
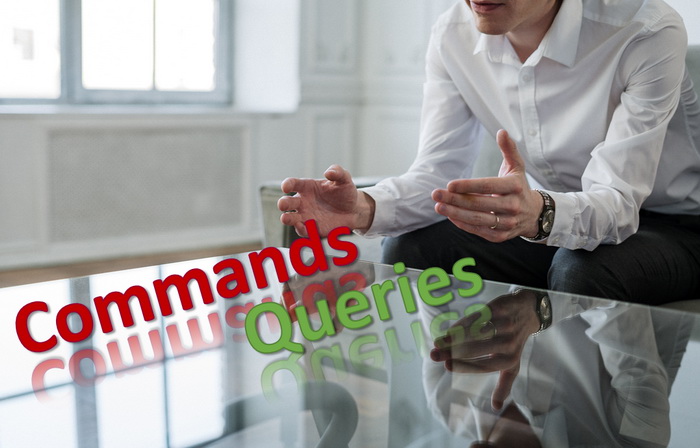
The purpose of this article is not to criticize the MediatR library. MediatR is a tool - and just like any tool, it has its own scope of application, and being used incorrectly might do more harm than good. This blog post summarizes my thoughts about using MediatR for supporting CQRS architecture.
... Read More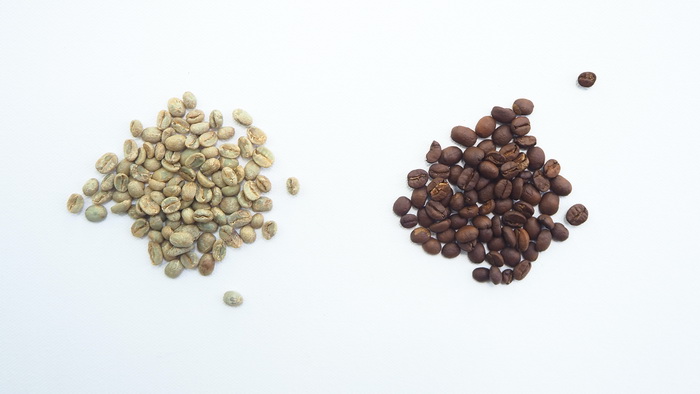
In this blog post, I’m going to share my experience on testing ASP.NET Core
applications by applying an unconventional method called snapshot assertions
. In comparison to the classical approach, this method should save you a lot of time and improve assertions maintainability.
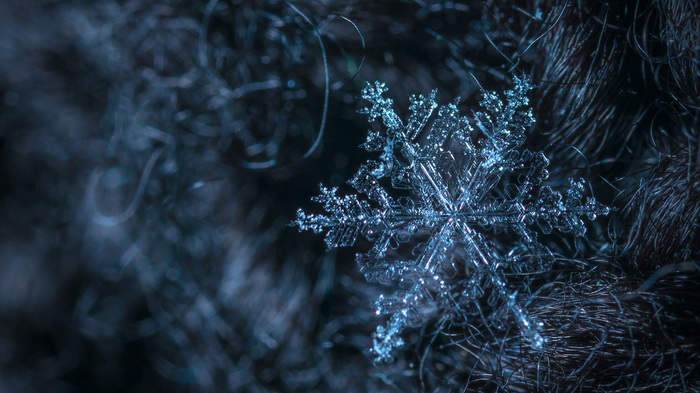
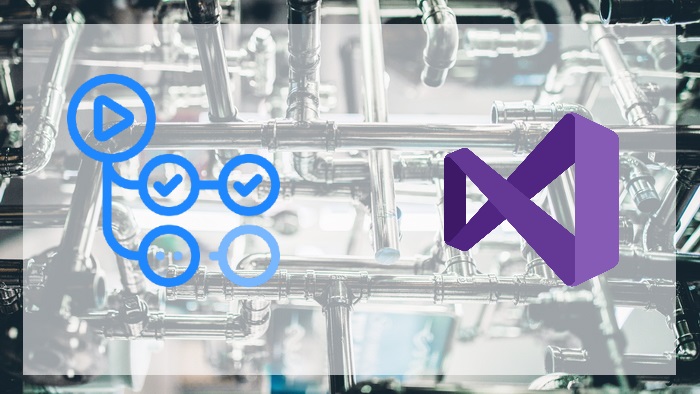
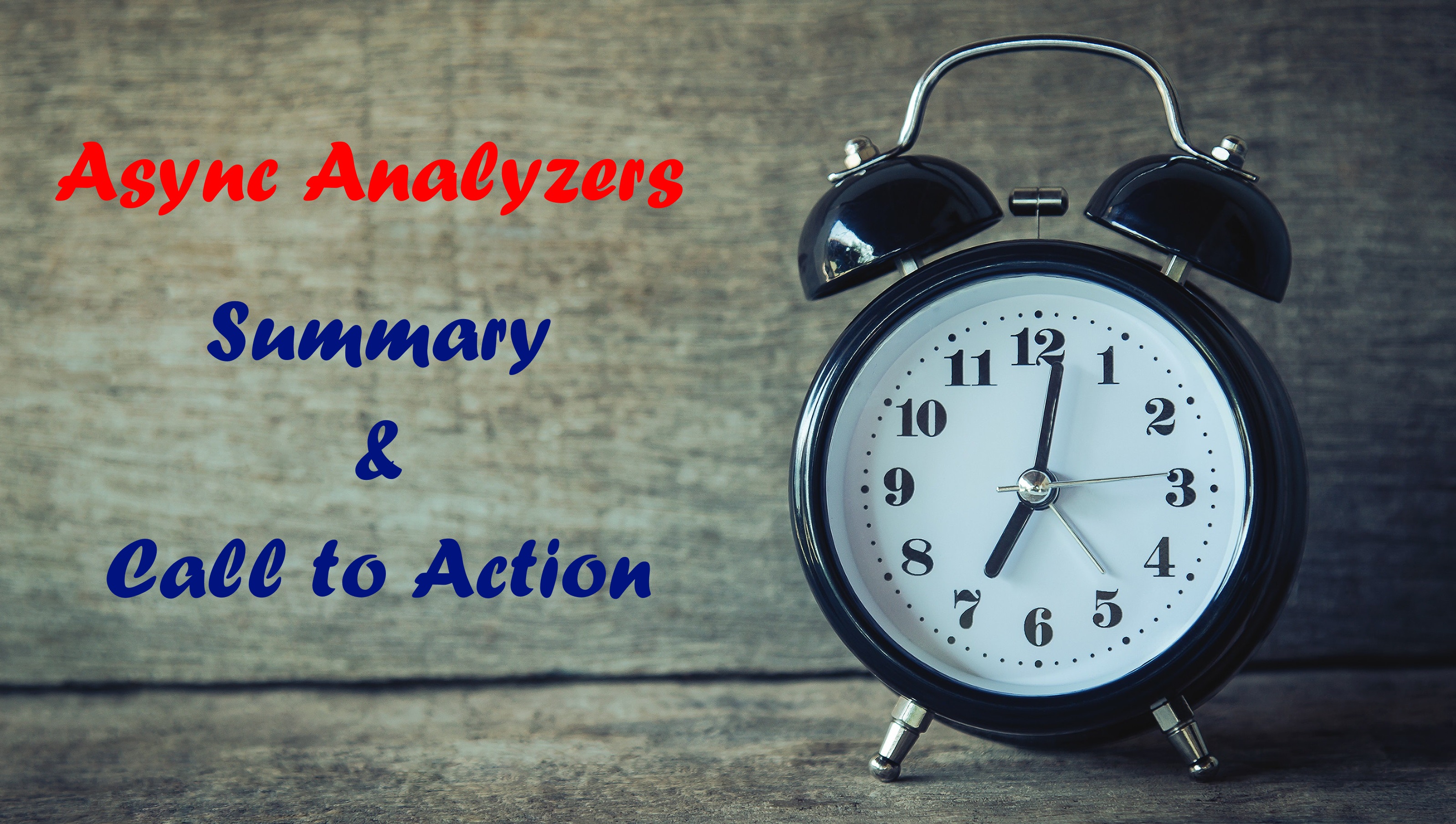
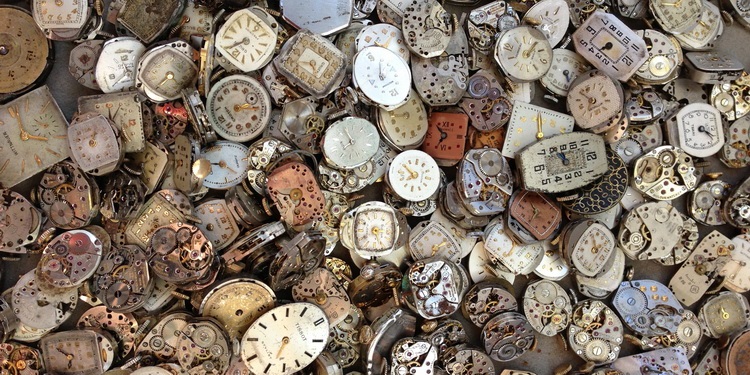
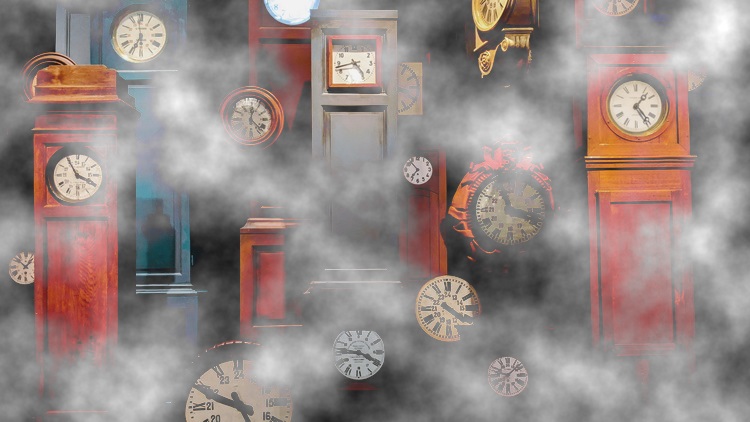
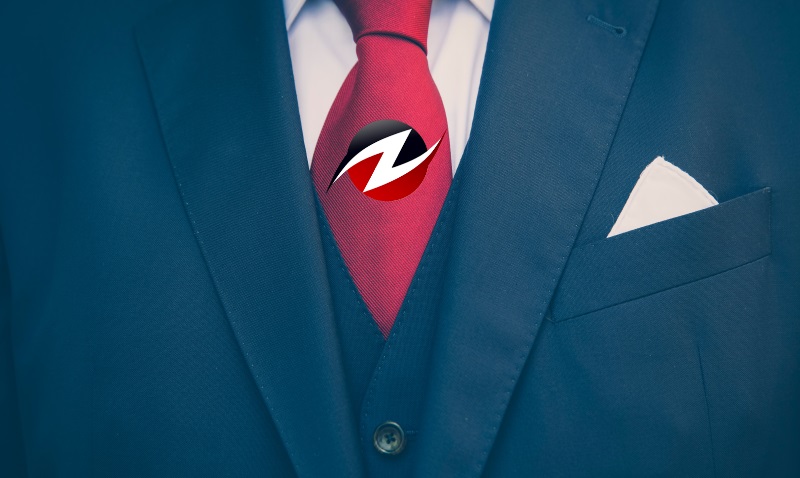
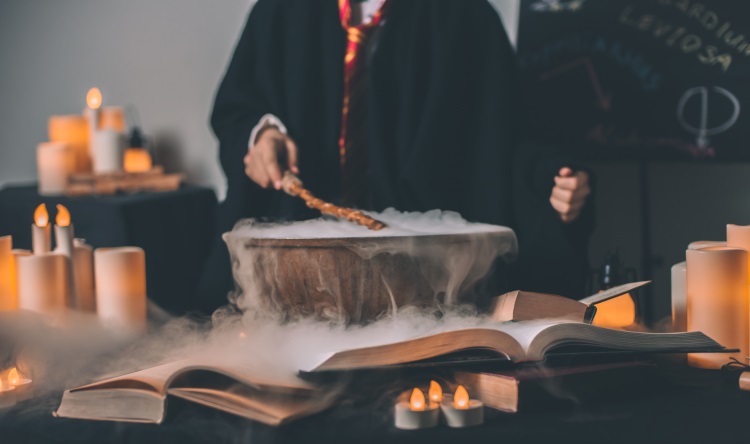
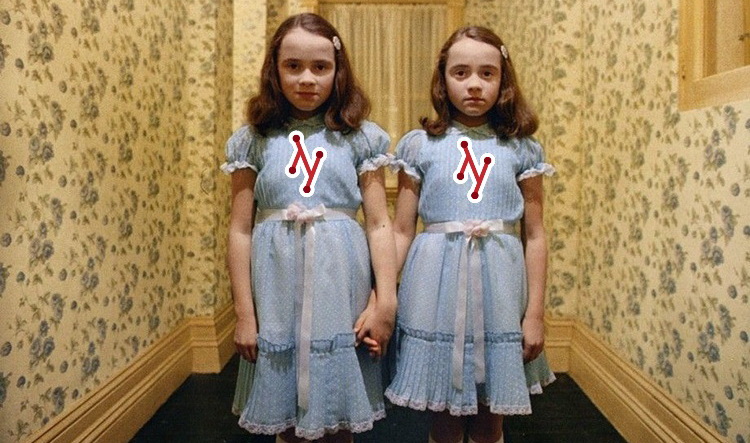
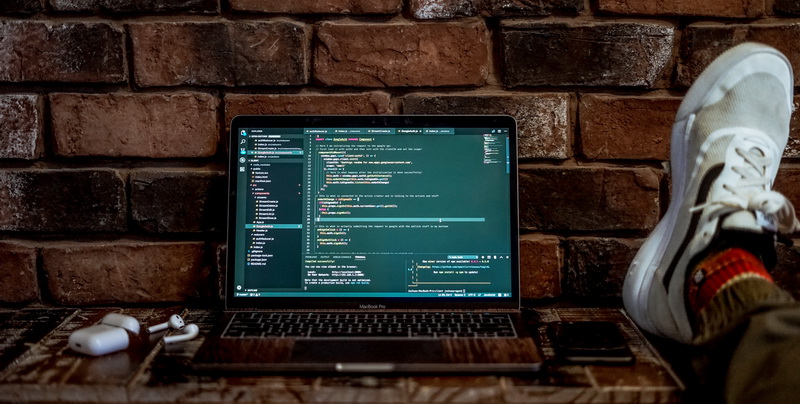
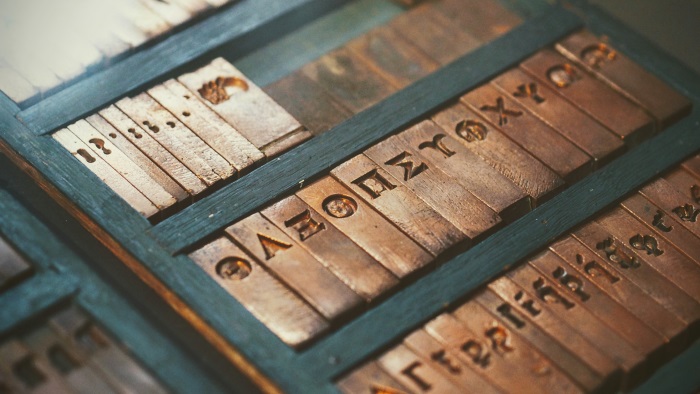
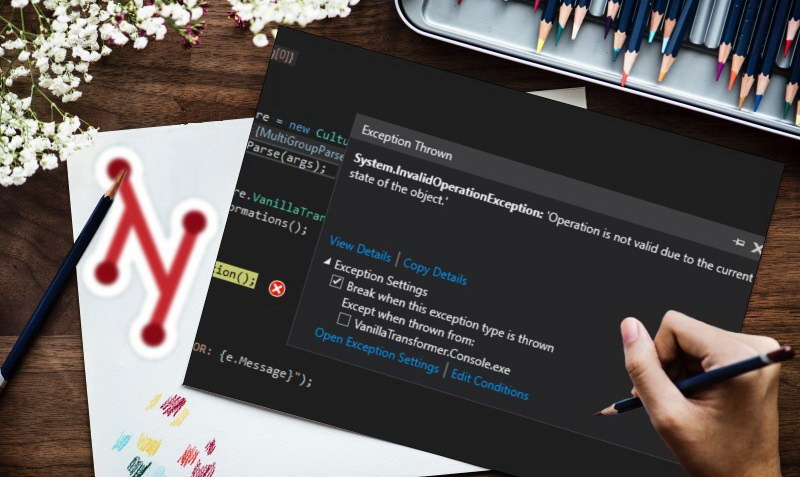
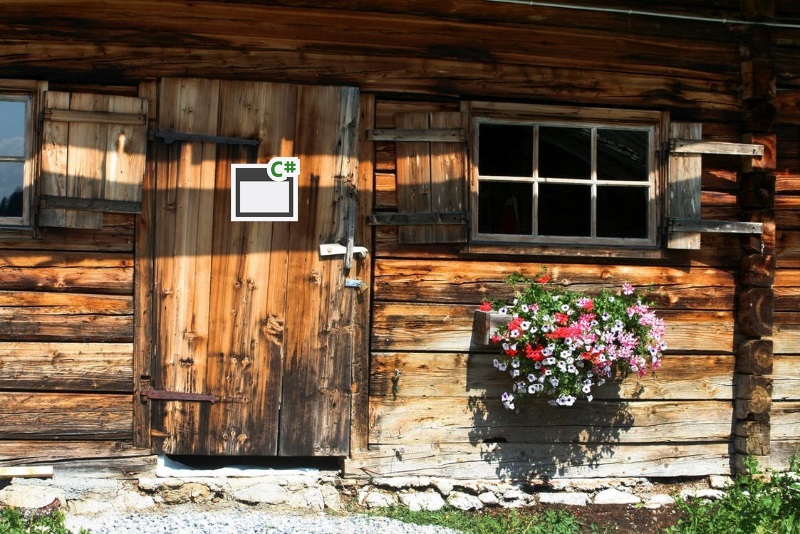
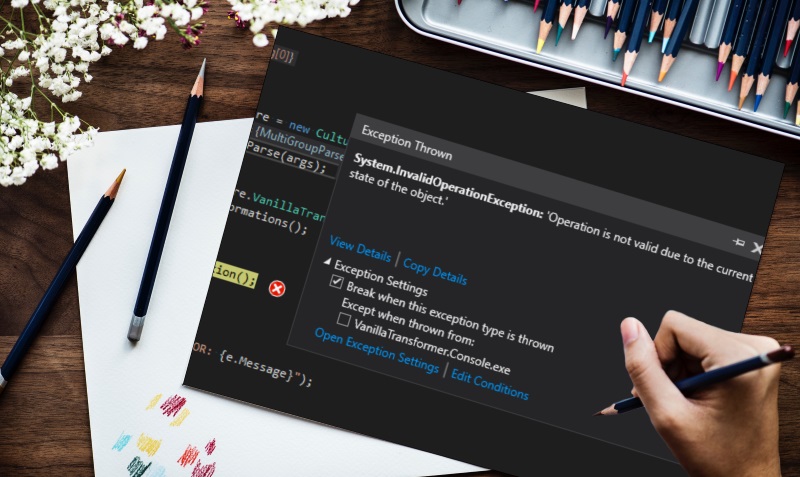
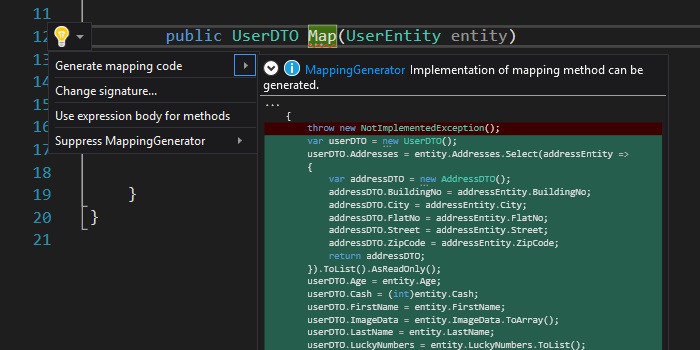
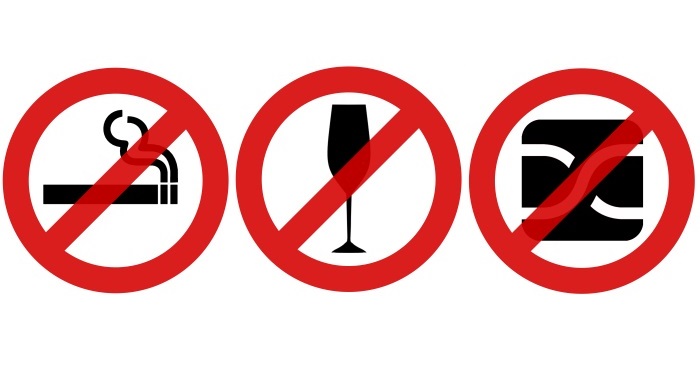