Articles about: csharp
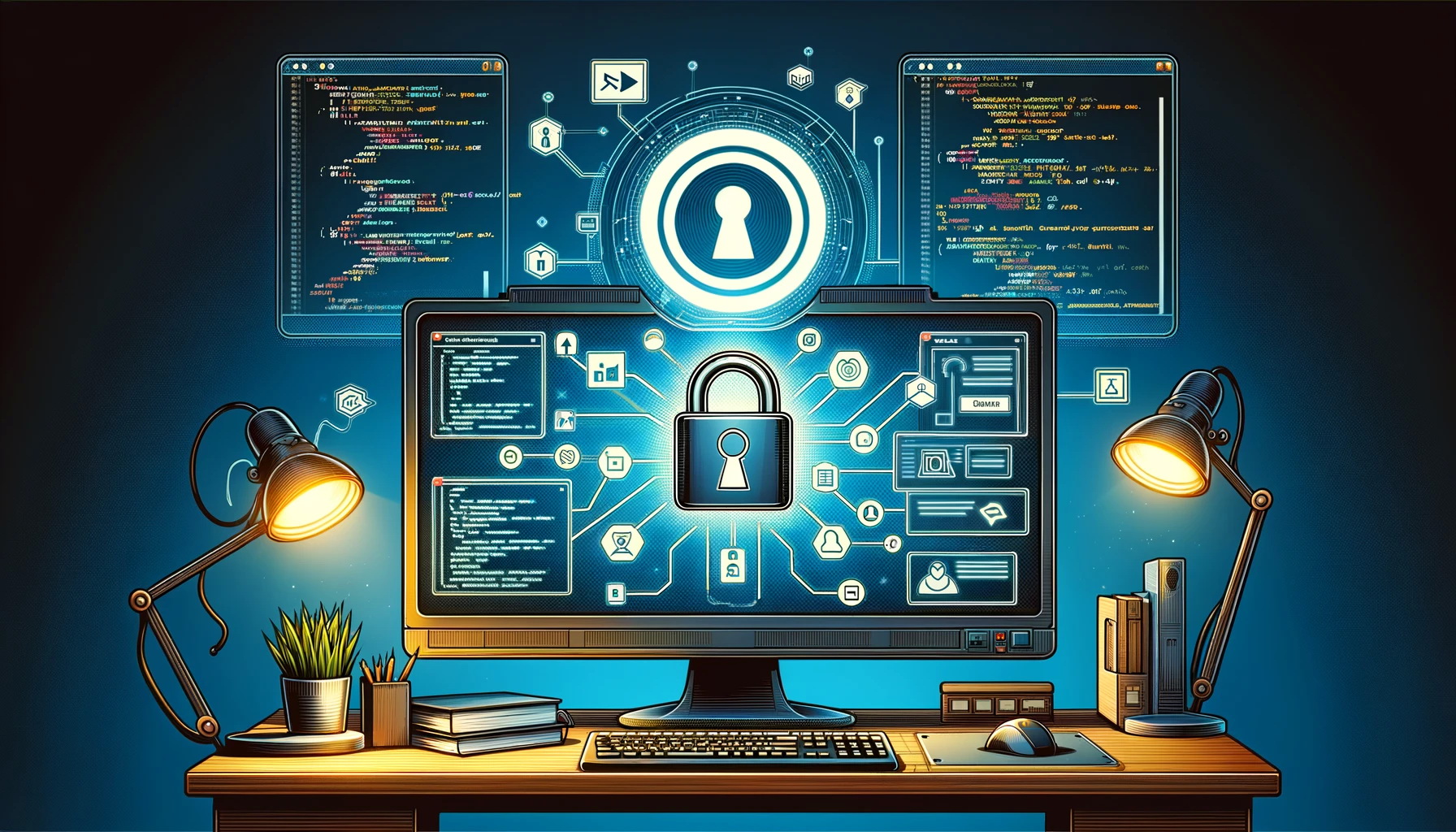
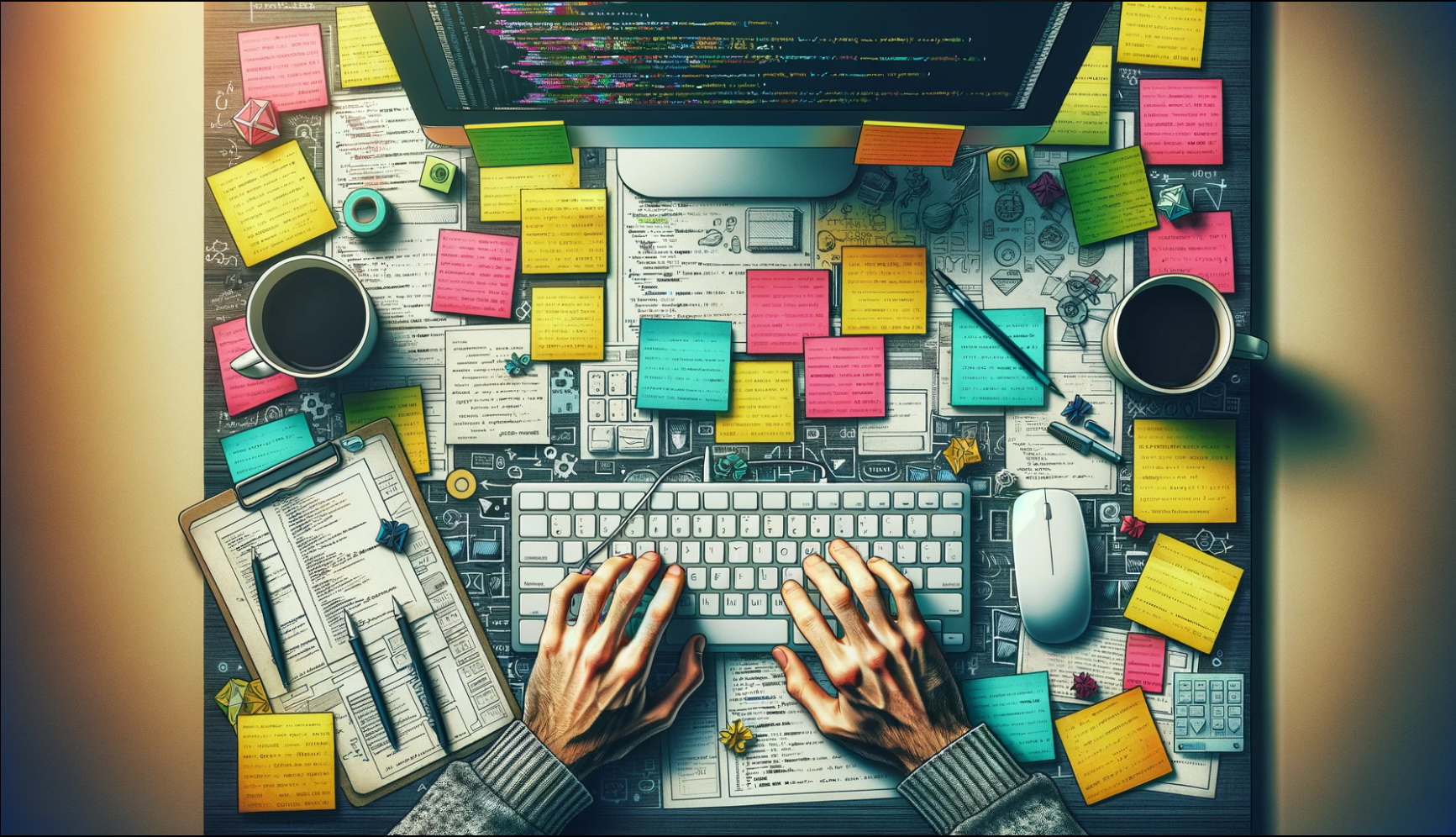
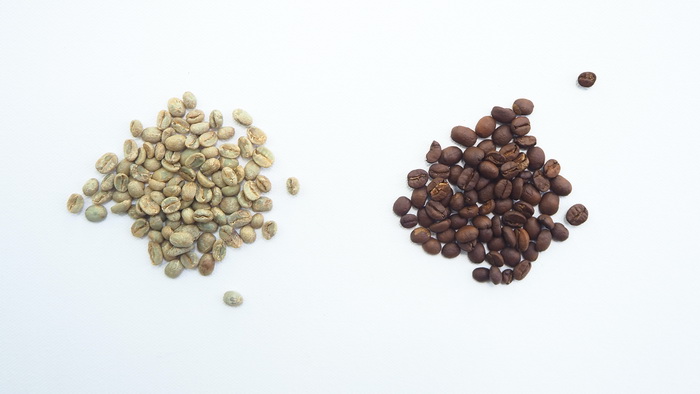
In this blog post, I’m going to share my experience on testing ASP.NET Core
applications by applying an unconventional method called snapshot assertions
. In comparison to the classical approach, this method should save you a lot of time and improve assertions maintainability.
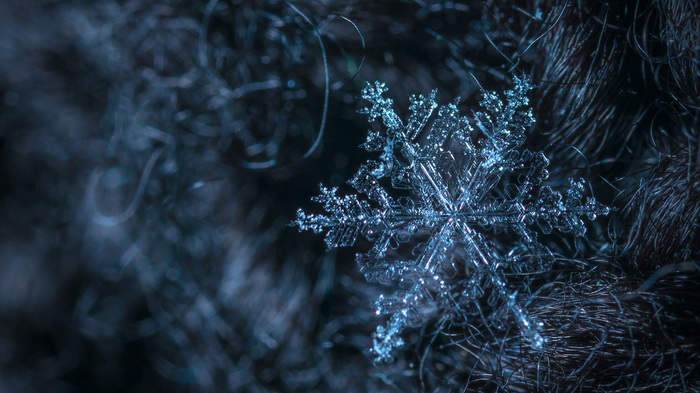
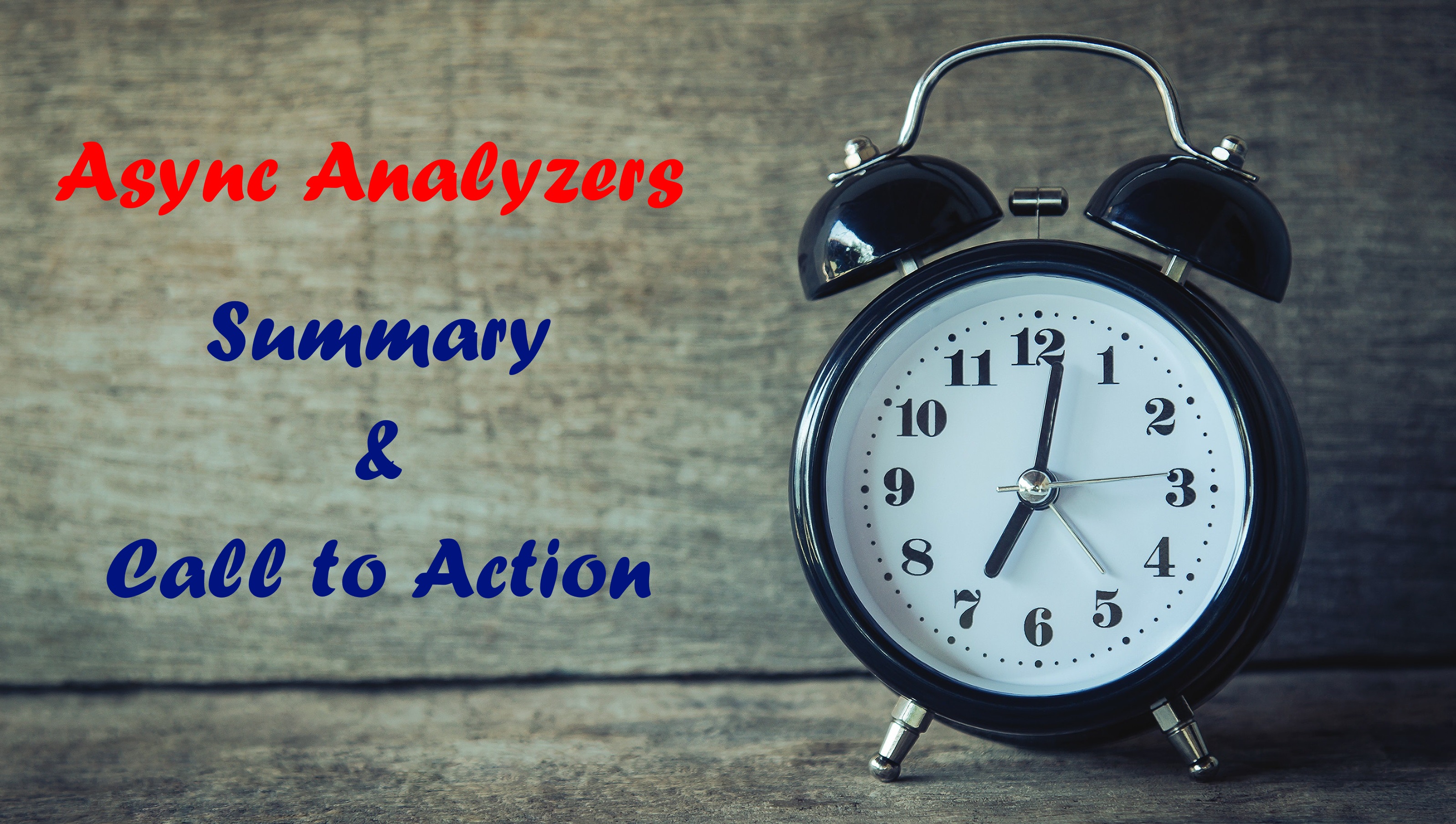
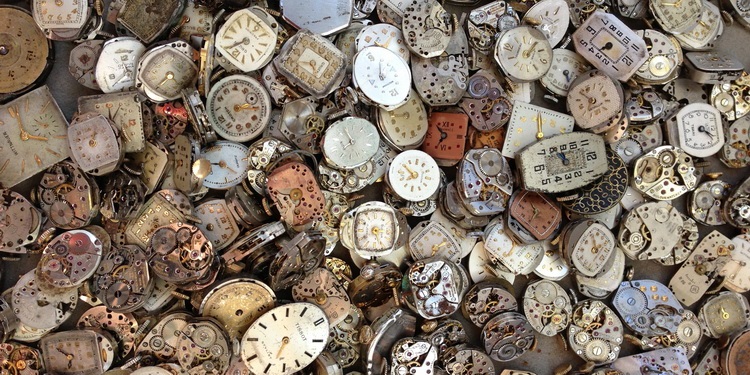
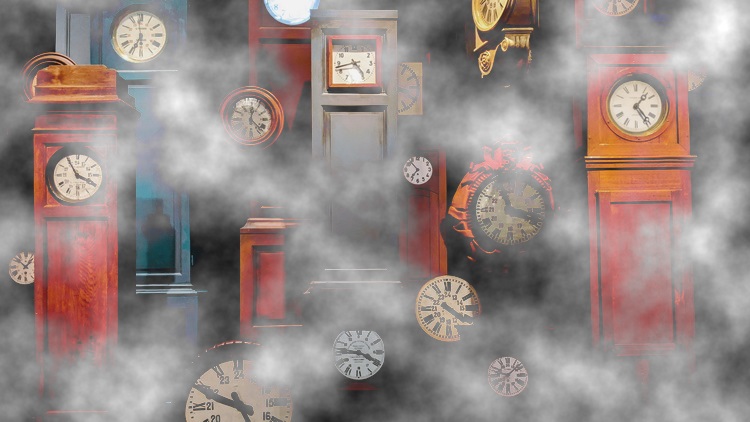
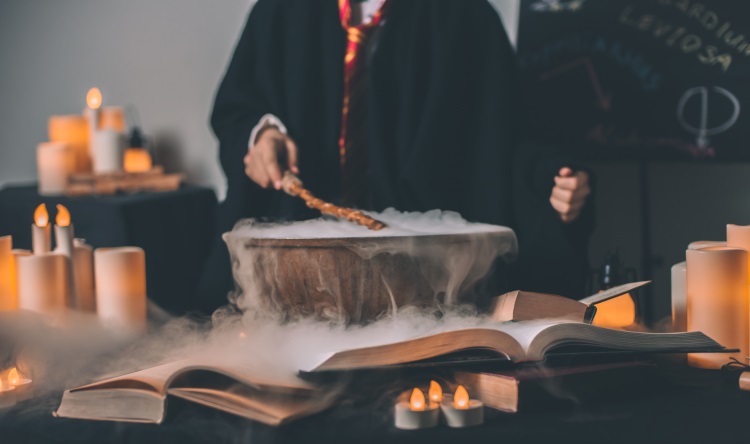
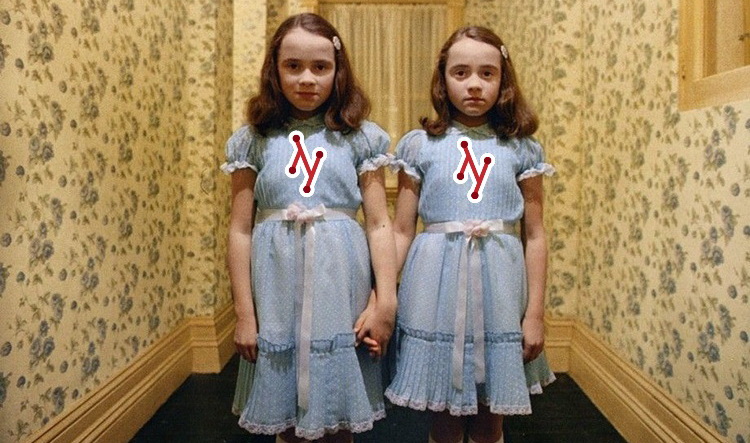
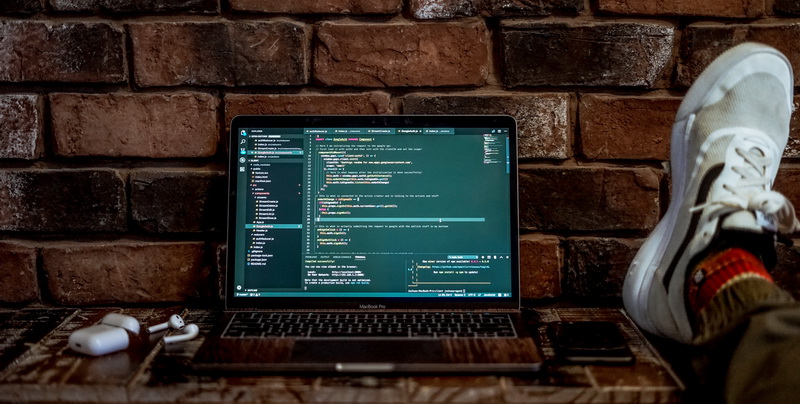
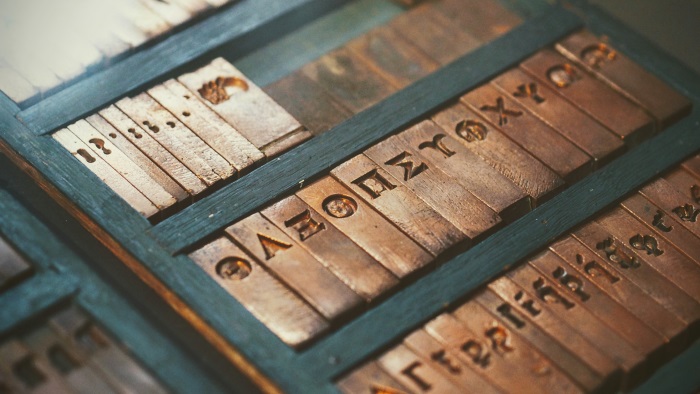
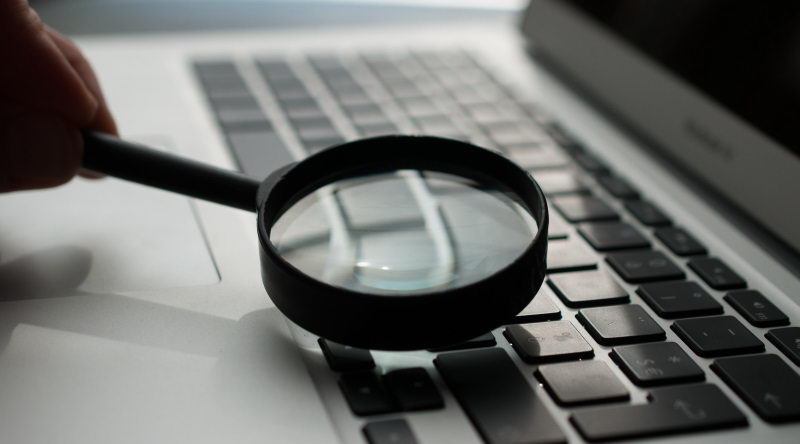