Immutable types in C# with Roslyn
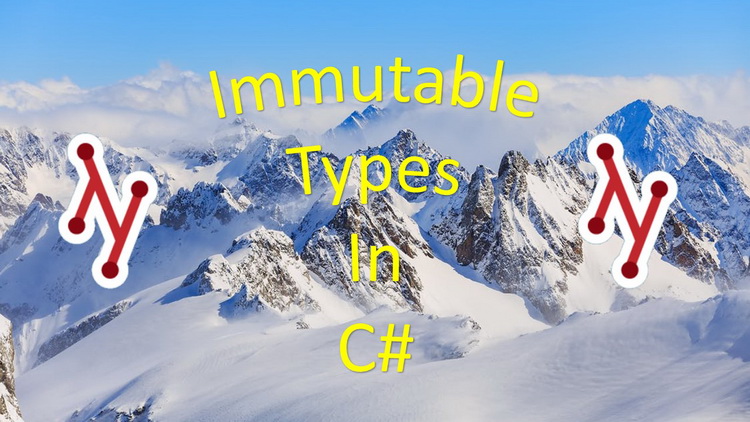
Some time ago I came across Jimmy Bogard’s article “Immutability in DTOs?” about the pros and cons of using immutable type pattern/approach. I fully agree with the author - the idea of immutable types is great but without the proper support from the language syntax it might not be worth applying. C# allows creating immutable types by adding readonly
keyword to fields or by removing setter from properties. We are obligated then to initialize those readonly members from the constructor or directly in the member’s definition. This results in a large amount of boilerplate code, causes problems with ORMs and serializers which require a default constructor, and makes the object instantiation cumbersome (or at least less readable). I’m a huge fan of Roslyn
so I’ve started thinking about how to utilize Roslyn’s API to avoid all those problems with immutable types and improve coding experience while working with them. In this article, I’m going to present the results of my experiments with Roslyn analyzers that simulate types immutability.
Convenient initialization 🔗︎
As I’ve mentioned before, in order to make a class immutable we need to remove setters, add a dedicated constructor for initializing those properties and then always use this constructor to instantiate our immutable class. A sample code snippet just to remind you how cumbersome it is:
public class UserDTO
{
public string FirstName { get;}
public string LastName { get;}
public int Age { get; }
public UserDTO(string firstName, string lastName, int age)
{
FirstName = firstName;
LastName = lastName;
Age = age;
}
}
class Program
{
static void Main(string[] args)
{
var user = new UserDTO("John", "Doe", 20);
}
}
It would be more convenient if we didn’t need to define a constructor and initialize members using the initialization block. However, there’s no mechanism that allows enforcing mandatory initialization in the init block. So, let’s introduce [InitRequired]
attribute inspired by initonly
keyword from C# records proposal:
If we want to enforce mandatory initialization via initialization block for all members, we can mark our type with [InitRequired]
attribute.
Of course, the property to be able to initialize via init block it must meet certain conditions:
- must have a setter
- the setter needs to be available in a given context (accessibility)
- cannot be a part of explicit interface implementation.
In order to avoid missing initialization caused by the conditions mentioned above, I would recommend always keeping those properties on the same accessibility level as the containing type.
Pro Tip: You can use MappingGenerator to complete initialization block with local accessible values
or to scaffold this initialization with sample values
![]()
If you don’t have access to the source code or you want to enforce full initialization only for given instance, you can do that by adding /*FullInitRequired*/
comment marker:
To ensure that full object graph is initialized, use /*FullInitRequired:recursive*/
comment marker. I think this may be especially useful for methods performing mapping or deep clone. I got the idea of those comment markers from a discussion about String Hints
#2796. This kind of annotation is already used for marking string literals with regex pattern:
Full immutability 🔗︎
[InitRequired]
attribute enforces only mandatory initialization via initialization block. To achieve immutability, we need to forbid modification outside the init block. For that purpose, I’ve introduced [InitOnly]
attribute. Basically, it works in the same way as [InitRequired]
but additionally it verifies if members decorated with it are not modified after initialization.
You can enforce immutability for all members by putting [InitOnly]
attribute on the type level.
Thanks to [InitOnly]
attribute and corresponding analyzer we can achieve full immutability without writing redundant boilerplate code.
Important: If you like the idea of
[InitRequire]
and[InitOnly]
attributes and you are going to use them in your project, please make sure that all your teammates know about it.
Summary 🔗︎
All attributes and analyzers described here are available as a single Nuget package SmartAnalyzers.CSharpExtensions.Annotations. The source code is published on Github
under CSharpExtensions project. Please let me know what you think about those extensions to C# language and if you encounter any problems with using it, feel free to report an issue on Github page.
UPDATE 2020-03-17: I added support for non-nullable references. Since now, the compiler should stop reporting "CS8618: Non-nullable property ‘PropertyName’ is uninitialized"
diagnostic for all members affected by [InitRequired]
and [InitOnly]
attributes.
UPDATE 2020-06-20: After the feature request #9 from Avi Levin (@Arithmomaniac) I decided to add [InitOnlyOptional]
attribute which works in similar way like [InitOnly]
but allows to make given field optional in initialization block. Analyzers for this new attribute verify if:
- property/field declaration provides a default value
- property/field is not modified outside the initialization block
Example usage:
public class Finance
{
//default value is required
//default value can be overridden only via the initialization block
[InitOnlyOptional]
public int Profit { get; set; } = 45;
}
UPDATE 2020-06-20: There’s a hope that init-only
concept will get a language-level support in C# 9. You can read more about that in the article Welcome to C# 9.0